Sustainability
Meets Real-World Action
5ire revolutionizes blockchain, prioritizing impact and sustainability. It bridges blockchain with real-world entities, fostering transformative development.
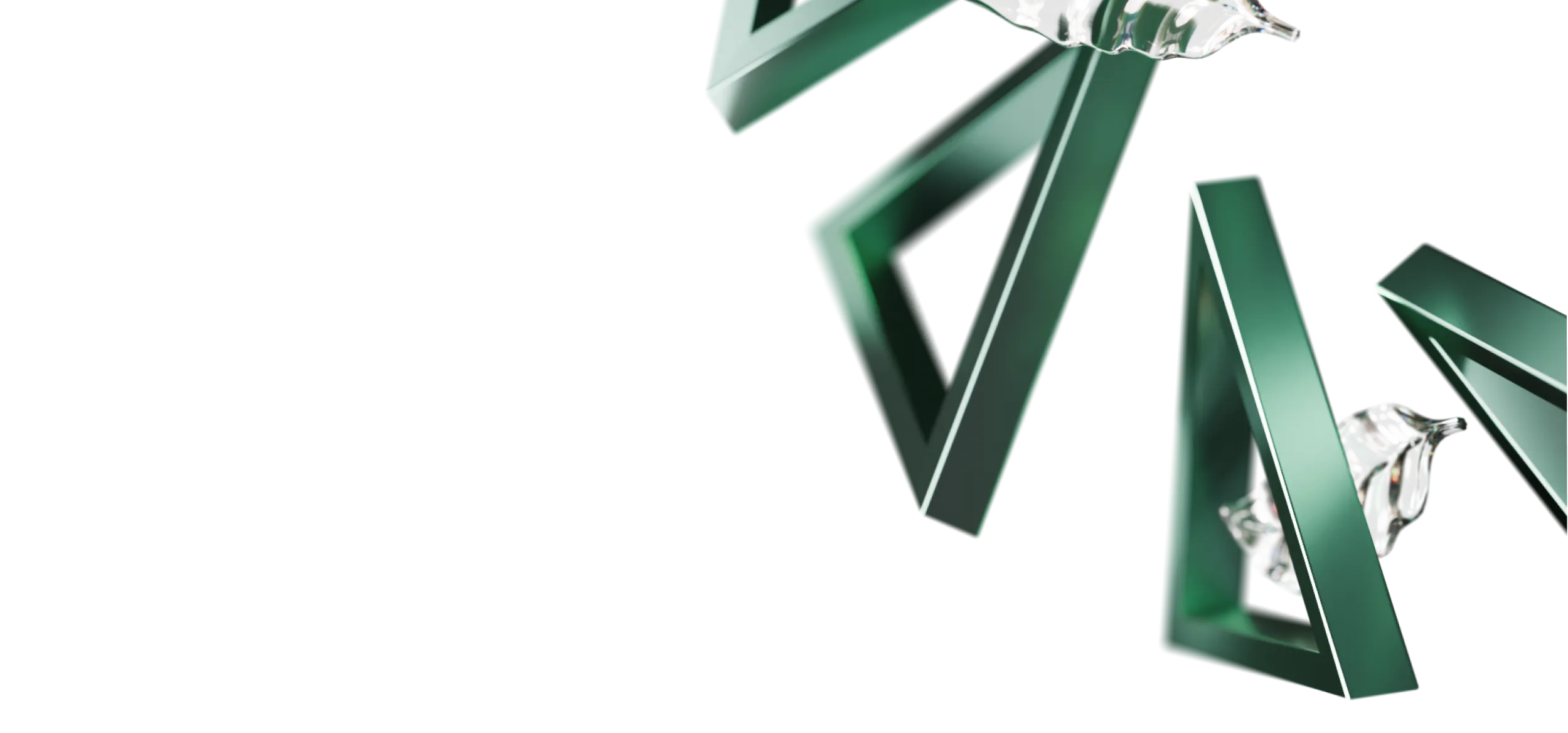
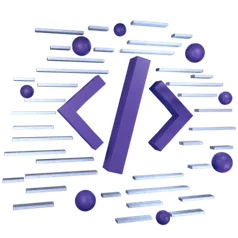
For Developers
Start building your next project on sustainable blockchain platform - 5ireChain.
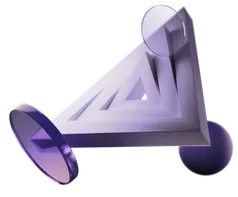
For Validators
Participate in our cause to sustainability by running a 5ireChain node.
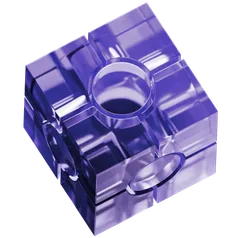
Research
Review and Analyze our research on the use of decentralized technology to enable sustainable solutions.
About 5ire
Brand Assets
FAQ
Join the Community
Developer Relation
Terms & Condition